Getting Started with the Helpdesk API
The UserVoice Helpdesk API exposes the core end-user and admin functionality of UserVoice to make it easy for you to build client applications or integrations with your own systems.
1. Create an API client
Before you can make any requests you need to create an API client. Go to your UserVoice Admin Console.
Go to Settings → Integrations → Helpdesk API, and click Add API Client.
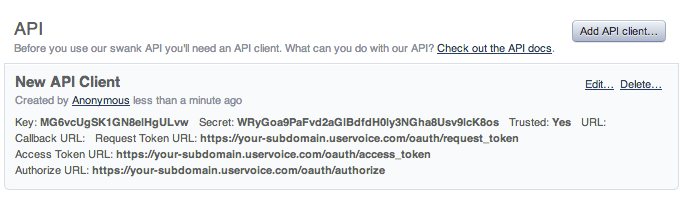
Enter a name for the API Client and then decide if you want the client to be trusted or not. Some endpoints require a trusted client. However, DO NOT check this if your API Key will be stored in an insecure environment (for example: a Javascript client application that end-users download)!
2. Install the SDK of your choice
To make one of the requests in our API Reference, you can use one of our API clients depending on the programming language you plan to use.
The UserVoice C# library can be installed using NuGet, the package manager. Follow the instructions in NuGet Gallery and run this command in Package Manager Console:
1
|
|
Place the following in your pom.xml when using Maven:
1 2 3 4 5 |
|
Remember to check the latest version!
Then fetch the package with all the dependencies by running:
1
|
|
The PHP library uses Composer as a package manager and requires two extensions to be installed and then loaded in php.ini:
- OAuth: Installing/Configuring. Homebrew: brew install php54-oauth
- mcrypt: Installing/Configuring. Homebrew: brew install php54-mcrypt
After installing OAuth and mcrypt, make sure the extensions are loaded from your php.ini:
1 2 |
|
Composer requires you to create a dependency file composer.json, where you include uservoice/uservoice as a dependency:
1 2 3 4 5 |
|
Now execute the dependency manager (installation) in your project root:
1
|
|
1 2 3 4 5 6 |
|
Install the uservoice Python module from PyPI:
1
|
|
or download the latest release from PyPI, unpack it and use the setup.py method:
1 2 |
|
Add this into your Gemfile:
1
|
|
And run Bundler:
1
|
|
If you’re not using Bundler, you can install it gem for the SDK like this:
1
|
|
3. Make an API request
Alright! You’re almost ready. Now, Get the API KEY and API SECRET from the API client you created in step one and replace the corresponding subdomain name with your UserVoice subdomain.
Then you can make an example request as the account owner (provided that you created a Trusted client):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
1 2 3 4 5 6 7 8 9 10 |
|
1 2 3 4 5 6 7 8 9 10 11 |
|
Rate Limiting
API requests have per minute rate limiting. Each request counts toward your limit. In addition, in order to account for expensive endpoints that tax our servers more, if the request takes longer than 1 second to compute then another “request” is counted for every whole second after the first.
If you are above your limit your requests will return a 429 HTTP error response.
Every request returns the following HTTP headers:
- X-Rate-Limit-Limit: The amount of requests available every minute
- X-Rate-Limit-Remaining: The amount of requests remaining this period
- X-Rate-Limit-Reset: The unix epoch in seconds when the limit resets
The limit depends on your plan. See Terms of Service for details.